The Spine Runtimes are available for many programming languages. To simplify documentation, the API reference below is programming language agnostic. There may be minor differences for some languages, such as start caps for spine-csharp and class name prefixes on methods for spine-c.Parameters cannot be null unless explicitly mentioned. Returns values will not be null unless explicitly mentioned. This documentation is for the latest non-beta Spine Runtimes (4.2). Documentation for the latest beta Spine Runtimes is also available.
This diagram illustrates how the various pieces of the runtimes fit together. Click for full resolution.
The instance data is created for each skeleton instance and stores the skeleton's pose and other state. The setup pose data is stateless and shared across skeleton instances. It contains the setup pose, attachments, and animations.
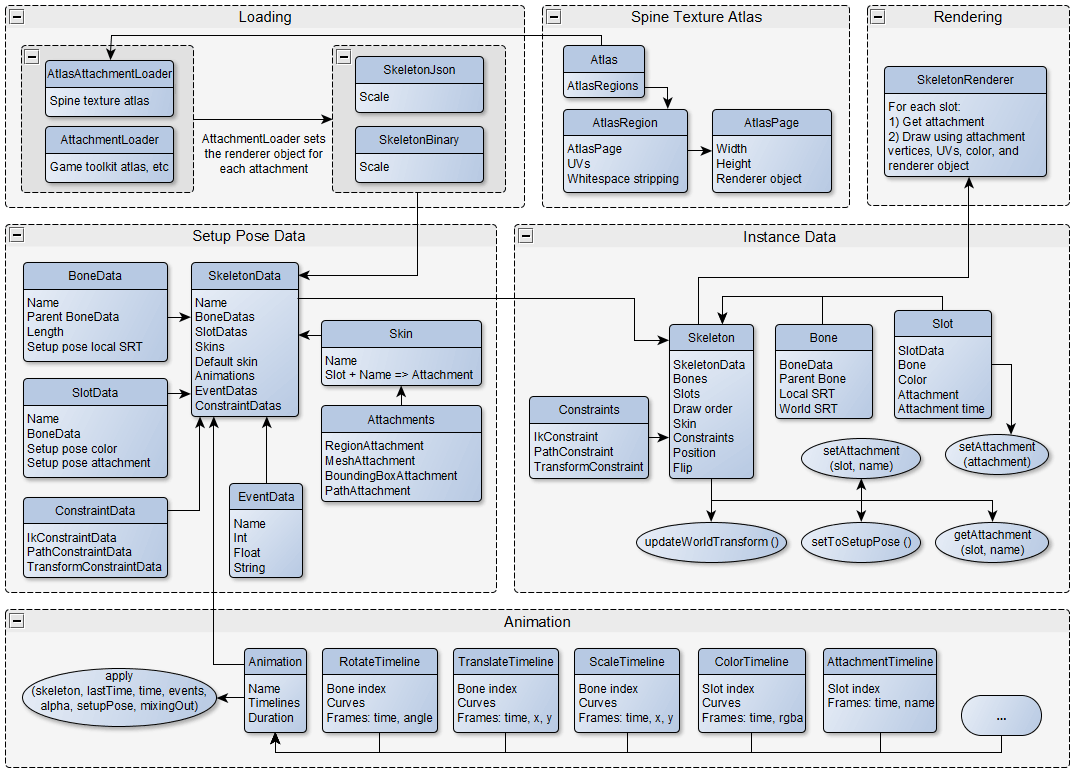
Stores a list of timelines to animate a skeleton's pose over time.
- Animation Properties
- duration: float
- The duration of the animation in seconds, which is usually the highest time of all frames in the timeline. The duration is used to know when it has completed and when it should loop back to the start.
- name: string readonly
- The animation's name, which is unique across all animations in the skeleton.
- timelines: list<Timeline>
- If the returned array or the timelines it contains are modified, timelines must be called.
- Animation Methods
apply ( Skeleton skeleton, float lastTime, float time, bool loop, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void - Applies the animation's timelines to the specified skeleton. See Timeline apply.
skeleton
The skeleton the animation is being applied to. This provides access to the bones, slots, and other skeleton components the timelines may change.lastTime
The last time in seconds this animation was applied. Some timelines trigger only at specific times rather than every frame. Pass -1 the first time an animation is applied to ensure frame 0 is triggered.time
The time in seconds the skeleton is being posed for. Most timelines find the frame before and the frame after this time and interpolate between the frame values. If beyond the duration andloop
is true then the animation will repeat, else the last frame will be applied.loop
If true, the animation repeats after the duration.events
If any events are fired, they are added to this list. Can be null to ignore fired events or if no timelines fire events.alpha
0 applies the current or setup values (depending onblend
). 1 applies the timeline values. Between 0 and 1 applies values between the current or setup values and the timeline values. By adjustingalpha
over time, an animation can be mixed in or out.alpha
can also be useful to apply animations on top of each other (layering).blend
Controls how mixing is applied whenalpha
< 1.direction
Indicates whether the timelines are mixing in or out. Used by timelines which perform instant transitions, such as DrawOrderTimeline or AttachmentTimeline.
hasTimeline ( string[] propertyIds): bool - Returns true if this animation contains a timeline with any of the specified property IDs.
The base class for all timelines.
- Timeline Properties
- duration: float readonly
- frameCount: int readonly
- The number of frames for this timeline.
- frameEntries: int readonly
- The number of entries stored per frame.
- frames: float[] readonly
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- Timeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void - Applies this timeline to the skeleton.
skeleton
The skeleton to which the timeline is being applied. This provides access to the bones, slots, and other skeleton components that the timeline may change.lastTime
The last time in seconds this timeline was applied. Timelines such as EventTimeline trigger only at specific times rather than every frame. In that case, the timeline triggers everything betweenlastTime
(exclusive) andtime
(inclusive). Pass -1 the first time an animation is applied to ensure frame 0 is triggered.time
The time in seconds that the skeleton is being posed for. Most timelines find the frame before and the frame after this time and interpolate between the frame values. If beyond the last frame, the last frame will be applied.events
If any events are fired, they are added to this list. Can be null to ignore fired events or if the timeline does not fire events.alpha
0 applies the current or setup value (depending onblend
). 1 applies the timeline value. Between 0 and 1 applies a value between the current or setup value and the timeline value. By adjustingalpha
over time, an animation can be mixed in or out.alpha
can also be useful to apply animations on top of each other (layering).blend
Controls how mixing is applied whenalpha
< 1.direction
Indicates whether the timeline is mixing in or out. Used by timelines which perform instant transitions, such as DrawOrderTimeline or AttachmentTimeline, and others such as ScaleTimeline.
Changes the alpha for a slot's color.
- AlphaTimeline Properties
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- slotIndex: int readonly
- AlphaTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a slot's attachment.
- AttachmentTimeline Properties
- attachmentNames: string[] readonly
- The attachment name for each frame. May contain null values to clear the attachment.
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- slotIndex: int readonly
- AttachmentTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
setFrame ( int frame, float time, string attachmentName): void - Sets the time and attachment name for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
An interface for timelines which change the property of a bone.
- BoneTimeline Properties
- boneIndex: int readonly
- The index of the bone in bones that will be changed when this timeline is applied.
CurveTimeline
extends TimelineThe base class for timelines that interpolate between frame values using stepped, linear, or a Bezier curve.
- CurveTimeline Properties
- LINEAR = 0: int static, readonly
- STEPPED = 1: int static, readonly
- BEZIER = 2: int static, readonly
- BEZIER_SIZE = 18: int static, readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- CurveTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getBezierValue ( float time, int frameIndex, int valueOffset, int i): float - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int - Returns the interpolation type for the specified frame.
setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.
bezier
The ordinal of this Bezier curve for this timeline, between 0 andbezierCount - 1
(specified in the constructor), inclusive.frame
Between 0 andframeCount - 1
, inclusive.value
The index of the value for the frame this curve is used for.time1
The time for the first key.value1
The value for the first key.cx1
The time for the first Bezier handle.cy1
The value for the first Bezier handle.cx2
The time of the second Bezier handle.cy2
The value for the second Bezier handle.time2
The time for the second key.value2
The value for the second key.
setLinear ( int frame): void - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
CurveTimeline1
extends CurveTimelineThe base class for a CurveTimeline that sets one property.
- CurveTimeline1 Properties
- ENTRIES = 2: int static, readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- CurveTimeline1 Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
CurveTimeline2
extends CurveTimelineThe base class for a CurveTimeline which sets two properties.
- CurveTimeline2 Properties
- ENTRIES = 3: int static, readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- CurveTimeline2 Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value1, float value2): void - Sets the time and values for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a slot's deform to deform a VertexAttachment.
- DeformTimeline Properties
- attachment: VertexAttachment readonly
- The attachment that will be deformed. See timelineAttachment.
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- slotIndex: int readonly
- vertices: float[][] readonly
- The vertices for each frame.
- DeformTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float[] vertices): void - Sets the time and vertices for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.vertices
Vertex positions for an unweighted VertexAttachment, or deform offsets if it has weights.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
DrawOrderTimeline
extends TimelineChanges a skeleton's drawOrder.
- DrawOrderTimeline Properties
- drawOrders: int[][] readonly
- The draw order for each frame. See setFrame.
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- DrawOrderTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
setFrame ( int frame, float time, int[] drawOrder): void - Sets the time and draw order for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.drawOrder
For each slot in slots, the index of the slot in the new draw order. May be null to use setup pose draw order.
EventTimeline
extends TimelineFires an Event when specific animation times are reached.
- EventTimeline Properties
- duration: float readonly, from Timeline
- events: Event[] readonly
- The event for each frame.
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- EventTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Fires events for frames >
lastTime
and <=time
.See Timeline apply. setFrame ( int frame, Event event): void - Sets the time and event for the specified frame.
frame
Between 0 andframeCount
, inclusive.
Changes a bone's inherit.
- InheritTimeline Properties
- ENTRIES = 2: int static, readonly
- boneIndex: int readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- InheritTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
setFrame ( int frame, float time, Inherit inherit): void - Sets the transform mode for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
IkConstraintTimeline
extends CurveTimeline- IkConstraintTimeline Properties
- ENTRIES = 6: int static, readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- ikConstraintIndex: int readonly
- The index of the IK constraint in ikConstraints that will be changed when this timeline is applied.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- IkConstraintTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float mix, float softness, int bendDirection, bool compress, bool stretch): void - Sets the time, mix, softness, bend direction, compress, and stretch for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.bendDirection
1 or -1.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
- PathConstraintMixTimeline Properties
- ENTRIES = 4: int static, readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- pathConstraintIndex: int readonly
- The index of the path constraint in pathConstraints that will be changed when this timeline is applied.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- PathConstraintMixTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float mixRotate, float mixX, float mixY): void - Sets the time and color for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a path constraint's position.
- PathConstraintPositionTimeline Properties
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- pathConstraintIndex: int readonly
- The index of the path constraint in pathConstraints that will be changed when this timeline is applied.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- PathConstraintPositionTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a path constraint's spacing.
- PathConstraintSpacingTimeline Properties
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- pathConstraintIndex: int readonly
- The index of the path constraint in pathConstraints that will be changed when this timeline is applied.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- PathConstraintSpacingTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a physics constraint's damping.
- PhysicsConstraintDampingTimeline Properties
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- physicsConstraintIndex: int readonly, from PhysicsConstraintTimeline
- The index of the physics constraint in physicsConstraints that will be changed when this timeline is applied, or -1 if all physics constraints in the skeleton will be changed.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- PhysicsConstraintDampingTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a physics constraint's gravity.
- PhysicsConstraintGravityTimeline Properties
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- physicsConstraintIndex: int readonly, from PhysicsConstraintTimeline
- The index of the physics constraint in physicsConstraints that will be changed when this timeline is applied, or -1 if all physics constraints in the skeleton will be changed.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- PhysicsConstraintGravityTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a physics constraint's inertia.
- PhysicsConstraintInertiaTimeline Properties
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- physicsConstraintIndex: int readonly, from PhysicsConstraintTimeline
- The index of the physics constraint in physicsConstraints that will be changed when this timeline is applied, or -1 if all physics constraints in the skeleton will be changed.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- PhysicsConstraintInertiaTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a physics constraint's massInverse. The timeline values are not inverted.
- PhysicsConstraintMassTimeline Properties
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- physicsConstraintIndex: int readonly, from PhysicsConstraintTimeline
- The index of the physics constraint in physicsConstraints that will be changed when this timeline is applied, or -1 if all physics constraints in the skeleton will be changed.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- PhysicsConstraintMassTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a physics constraint's mix.
- PhysicsConstraintMixTimeline Properties
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- physicsConstraintIndex: int readonly, from PhysicsConstraintTimeline
- The index of the physics constraint in physicsConstraints that will be changed when this timeline is applied, or -1 if all physics constraints in the skeleton will be changed.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- PhysicsConstraintMixTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Resets a physics constraint when specific animation times are reached.
- PhysicsConstraintResetTimeline Properties
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- physicsConstraintIndex: int readonly
- The index of the physics constraint in physicsConstraints that will be reset when this timeline is applied, or -1 if all physics constraints in the skeleton will be reset.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- PhysicsConstraintResetTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Resets the physics constraint when frames >
lastTime
and <=time
.See Timeline apply. setFrame ( int frame, float time): void - Sets the time for the specified frame.
frame
Between 0 andframeCount
, inclusive.
Changes a physics constraint's strength.
- PhysicsConstraintStrengthTimeline Properties
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- physicsConstraintIndex: int readonly, from PhysicsConstraintTimeline
- The index of the physics constraint in physicsConstraints that will be changed when this timeline is applied, or -1 if all physics constraints in the skeleton will be changed.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- PhysicsConstraintStrengthTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
The base class for most PhysicsConstraint timelines.
- PhysicsConstraintTimeline Properties
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- physicsConstraintIndex: int readonly
- The index of the physics constraint in physicsConstraints that will be changed when this timeline is applied, or -1 if all physics constraints in the skeleton will be changed.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- PhysicsConstraintTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a physics constraint's wind.
- PhysicsConstraintWindTimeline Properties
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- physicsConstraintIndex: int readonly, from PhysicsConstraintTimeline
- The index of the physics constraint in physicsConstraints that will be changed when this timeline is applied, or -1 if all physics constraints in the skeleton will be changed.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- PhysicsConstraintWindTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a slot's color.
- RGBATimeline Properties
- ENTRIES = 5: int static, readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- slotIndex: int readonly
- RGBATimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float r, float g, float b, float a): void - Sets the time and color for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
- RGBA2Timeline Properties
- ENTRIES = 8: int static, readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- slotIndex: int readonly
- The index of the slot in slots that will be changed when this timeline is applied. The darkColor must not be null.
- RGBA2Timeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float r, float g, float b, float a, float r2, float g2, float b2): void - Sets the time, light color, and dark color for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes the RGB for a slot's color.
- RGBTimeline Properties
- ENTRIES = 4: int static, readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- slotIndex: int readonly
- RGBTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float r, float g, float b): void - Sets the time and color for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
- RGB2Timeline Properties
- ENTRIES = 7: int static, readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- slotIndex: int readonly
- The index of the slot in slots that will be changed when this timeline is applied. The darkColor must not be null.
- RGB2Timeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float r, float g, float b, float r2, float g2, float b2): void - Sets the time, light color, and dark color for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a bone's local rotation.
- RotateTimeline Properties
- boneIndex: int readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- RotateTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
- ScaleTimeline Properties
- boneIndex: int readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- ScaleTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value1, float value2): void from CurveTimeline2 - Sets the time and values for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a bone's local scaleX.
- ScaleXTimeline Properties
- boneIndex: int readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- ScaleXTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a bone's local scaleY.
- ScaleYTimeline Properties
- boneIndex: int readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- ScaleYTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a slot's sequenceIndex for an attachment's Sequence.
- SequenceTimeline Properties
- ENTRIES = 3: int static, readonly
- attachment: Attachment readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- slotIndex: int readonly
- SequenceTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
setFrame ( int frame, float time, SequenceMode mode, int index, float delay): void - Sets the time, mode, index, and frame time for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
Seconds between frames.
- ShearTimeline Properties
- boneIndex: int readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- ShearTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value1, float value2): void from CurveTimeline2 - Sets the time and values for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a bone's local shearX.
- ShearXTimeline Properties
- boneIndex: int readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- ShearXTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a bone's local shearY.
- ShearYTimeline Properties
- boneIndex: int readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- ShearYTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
An interface for timelines which change the property of a slot.
- SlotTimeline Properties
- slotIndex: int readonly
- The index of the slot in slots that will be changed when this timeline is applied.
- TransformConstraintTimeline Properties
- ENTRIES = 7: int static, readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- transformConstraintIndex: int readonly
- The index of the transform constraint in transformConstraints that will be changed when this timeline is applied.
- TransformConstraintTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float mixRotate, float mixX, float mixY, float mixScaleX, float mixScaleY, float mixShearY): void - Sets the time, rotate mix, translate mix, scale mix, and shear mix for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
- TranslateTimeline Properties
- boneIndex: int readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- TranslateTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value1, float value2): void from CurveTimeline2 - Sets the time and values for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a bone's local x.
- TranslateXTimeline Properties
- boneIndex: int readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- TranslateXTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Changes a bone's local y.
- TranslateYTimeline Properties
- boneIndex: int readonly
- duration: float readonly, from Timeline
- frameCount: int readonly, from Timeline
- The number of frames for this timeline.
- frameEntries: int readonly, from Timeline
- The number of entries stored per frame.
- frames: float[] readonly, from Timeline
- The time in seconds and any other values for each frame.
- propertyIds: string[] readonly, from Timeline
- Uniquely encodes both the type of this timeline and the skeleton properties that it affects.
- TranslateYTimeline Methods
apply ( Skeleton skeleton, float lastTime, float time, list<Event> events, float alpha, MixBlend blend, MixDirection direction): void from Timeline - Applies this timeline to the skeleton.See Timeline apply.
getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup, float value): float from CurveTimeline1 getAbsoluteValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getBezierValue ( float time, int frameIndex, int valueOffset, int i): float from CurveTimeline - Returns the Bezier interpolated value for the specified time.
frameIndex
The index into frames for the values of the frame beforetime
.valueOffset
The offset fromframeIndex
to the value this curve is used for.i
The index of the Bezier segments. See getCurveType.
getCurveType ( int frame): int from CurveTimeline - Returns the interpolation type for the specified frame.
getCurveValue ( float time): float from CurveTimeline1 - Returns the interpolated value for the specified time.
getRelativeValue ( float time, float alpha, MixBlend blend, float current, float setup): float from CurveTimeline1 getScaleValue ( float time, float alpha, MixBlend blend, MixDirection direction, float current, float setup): float from CurveTimeline1 setBezier ( int bezier, int frame, int value, float time1, float value1, float cx1, float cy1, float cx2, float cy2, float time2, float value2): void from CurveTimeline - Stores the segments for the specified Bezier curve. For timelines that modify multiple values, there may be more than one curve per frame.See CurveTimeline setBezier.
setFrame ( int frame, float time, float value): void from CurveTimeline1 - Sets the time and value for the specified frame.
frame
Between 0 andframeCount
, inclusive.time
The frame time in seconds.
setLinear ( int frame): void from CurveTimeline - Sets the specified frame to linear interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
setStepped ( int frame): void from CurveTimeline - Sets the specified frame to stepped interpolation.
frame
Between 0 andframeCount - 1
, inclusive.
shrink ( int bezierCount): void from CurveTimeline - Shrinks the storage for Bezier curves, for use when
bezierCount
(specified in the constructor) was larger than the actual number of Bezier curves.
Controls how timeline values are mixed with setup pose values or current pose values when a timeline is applied with
alpha
< 1. See Timeline apply.- MixBlend Values
- setup
- Transitions from the setup value to the timeline value (the current value is not used). Before the first frame, the setup value is set.
- first
- Transitions from the current value to the timeline value. Before the first frame, transitions from the current value to the setup value. Timelines which perform instant transitions, such as DrawOrderTimeline or AttachmentTimeline, use the setup value before the first frame.
first
is intended for the first animations applied, not for animations layered on top of those. - replace
- Transitions from the current value to the timeline value. No change is made before the first frame (the current value is kept until the first frame).
replace
is intended for animations layered on top of others, not for the first animations applied. - add
- Transitions from the current value to the current value plus the timeline value. No change is made before the first frame (the current value is kept until the first frame).
add
is intended for animations layered on top of others, not for the first animations applied. Properties set by additive animations must be set manually or by another animation before applying the additive animations, else the property values will increase each time the additive animations are applied.
Indicates whether a timeline's
alpha
is mixing out over time toward 0 (the setup or current pose value) or mixing in toward 1 (the timeline's value). Some timelines use this to decide how values are applied. See Timeline apply.Applies animations over time, queues animations for later playback, mixes (crossfading) between animations, and applies multiple animations on top of each other (layering). See Applying Animations in the Spine Runtimes Guide.
- AnimationState Properties
- data: AnimationStateData
- The AnimationStateData to look up mix durations.
- timeScale: float
- Multiplier for the delta time when the animation state is updated, causing time for all animations and mixes to play slower or faster. Defaults to 1. See TrackEntry timeScale for affecting a single animation.
- tracks: list<TrackEntry> readonly
- The list of tracks that have had animations, which may contain null entries for tracks that currently have no animation.
- AnimationState Methods
addAnimation ( int trackIndex, string animationName, bool loop, float delay): TrackEntry - Queues an animation by name. See addAnimation.
addAnimation ( int trackIndex, Animation animation, bool loop, float delay): TrackEntry - Adds an animation to be played after the current or last queued animation for a track. If the track is empty, it is equivalent to calling setAnimation.
delay
If > 0, sets delay. If <= 0, the delay set is the duration of the previous track entry minus any mix duration (from the AnimationStateData) plus the specifieddelay
(ie the mix ends at (delay
= 0) or before (delay
< 0) the previous track entry duration). If the previous entry is looping, its next loop completion is used instead of its duration.<return>
A track entry to allow further customization of animation playback. References to the track entry must not be kept after the dispose event occurs.
addEmptyAnimation ( int trackIndex, float mixDuration, float delay): TrackEntry - Adds an empty animation to be played after the current or last queued animation for a track, and sets the track entry's mixDuration. If the track is empty, it is equivalent to calling setEmptyAnimation. See setEmptyAnimation.
delay
If > 0, sets delay. If <= 0, the delay set is the duration of the previous track entry minus any mix duration plus the specifieddelay
(ie the mix ends at (delay
= 0) or before (delay
< 0) the previous track entry duration). If the previous entry is looping, its next loop completion is used instead of its duration.<return>
A track entry to allow further customization of animation playback. References to the track entry must not be kept after the dispose event occurs.
addListener ( AnimationStateListener listener): void - Adds a listener to receive events for all track entries.
apply ( Skeleton skeleton): bool - Poses the skeleton using the track entry animations. The animation state is not changed, so can be applied to multiple skeletons to pose them identically.
<return>
True if any animations were applied.
clearListenerNotifications ( ): void - Discards all listener notifications that have not yet been delivered. This can be useful to call from an AnimationStateListener when it is known that further notifications that may have been already queued for delivery are not wanted because new animations are being set.
clearListeners ( ): void - Removes all listeners added with addListener.
clearNext ( TrackEntry entry): void - Removes the next and all entries after it for the specified entry.
clearTrack ( int trackIndex): void - Removes all animations from the track, leaving skeletons in their current pose. It may be desired to use setEmptyAnimation to mix the skeletons back to the setup pose, rather than leaving them in their current pose.
clearTracks ( ): void - Removes all animations from all tracks, leaving skeletons in their current pose. It may be desired to use setEmptyAnimations to mix the skeletons back to the setup pose, rather than leaving them in their current pose.
getCurrent ( int trackIndex): TrackEntry - Returns the track entry for the animation currently playing on the track, or null if no animation is currently playing.
<return>
May be null.
removeListener ( AnimationStateListener listener): void - Removes the listener added with addListener.
setAnimation ( int trackIndex, string animationName, bool loop): TrackEntry - Sets an animation by name. See setAnimation.
setAnimation ( int trackIndex, Animation animation, bool loop): TrackEntry - Sets the current animation for a track, discarding any queued animations. If the formerly current track entry was never applied to a skeleton, it is replaced (not mixed from).
loop
If true, the animation will repeat. If false it will not, instead its last frame is applied if played beyond its duration. In either case trackEnd determines when the track is cleared.<return>
A track entry to allow further customization of animation playback. References to the track entry must not be kept after the dispose event occurs.
setEmptyAnimation ( int trackIndex, float mixDuration): TrackEntry - Sets an empty animation for a track, discarding any queued animations, and sets the track entry's mixDuration. An empty animation has no timelines and serves as a placeholder for mixing in or out. Mixing out is done by setting an empty animation with a mix duration using either setEmptyAnimation, setEmptyAnimations, or addEmptyAnimation. Mixing to an empty animation causes the previous animation to be applied less and less over the mix duration. Properties keyed in the previous animation transition to the value from lower tracks or to the setup pose value if no lower tracks key the property. A mix duration of 0 still mixes out over one frame. Mixing in is done by first setting an empty animation, then adding an animation using addAnimation with the desired delay (an empty animation has a duration of 0) and on the returned track entry, set the setMixDuration. Mixing from an empty animation causes the new animation to be applied more and more over the mix duration. Properties keyed in the new animation transition from the value from lower tracks or from the setup pose value if no lower tracks key the property to the value keyed in the new animation.
setEmptyAnimations ( float mixDuration): void - Sets an empty animation for every track, discarding any queued animations, and mixes to it over the specified mix duration.
update ( float delta): void - Increments each track entry trackTime, setting queued animations as current if needed.
Stores mix (crossfade) durations to be applied when AnimationState animations are changed.
- AnimationStateData Properties
- defaultMix: float
- The mix duration to use when no mix duration has been defined between two animations.
- skeletonData: SkeletonData readonly
- The SkeletonData to look up animations when they are specified by name.
- AnimationStateData Methods
getMix ( Animation from, Animation to): float - Returns the mix duration to use when changing from the specified animation to the other, or the defaultMix if no mix duration has been set.
setMix ( Animation from, Animation to, float duration): void - Sets the mix duration when changing from the specified animation to the other. See mixDuration.
setMix ( string fromName, string toName, float duration): void - Sets a mix duration by animation name. See setMix.
The interface to implement for receiving TrackEntry events. It is always safe to call AnimationState methods when receiving events. TrackEntry events are collected during update and apply and fired only after those methods are finished. See TrackEntry listener and AnimationState addListener.
- AnimationStateListener Methods
complete ( TrackEntry entry): void - Invoked every time this entry's animation completes a loop. This may occur during mixing (after interrupt). If this entry's mixingTo is not null, this entry is mixing out (it is not the current entry). Because this event is triggered at the end of apply, any animations set in response to the event won't be applied until the next time the AnimationState is applied.
dispose ( TrackEntry entry): void - Invoked when this entry will be disposed. This may occur without the entry ever being set as the current entry. References to the entry should not be kept after
dispose
is called, as it may be destroyed or reused. end ( TrackEntry entry): void - Invoked when this entry will never be applied again. This only occurs if this entry has previously been set as the current entry (start was invoked).
event ( TrackEntry entry, Event event): void - Invoked when this entry's animation triggers an event. This may occur during mixing (after interrupt), see eventThreshold. Because this event is triggered at the end of apply, any animations set in response to the event won't be applied until the next time the AnimationState is applied.
interrupt ( TrackEntry entry): void - Invoked when another entry has replaced this entry as the current entry. This entry may continue being applied for mixing.
start ( TrackEntry entry): void - Invoked when this entry has been set as the current entry. end will occur when this entry will no longer be applied.
Stores settings and other state for the playback of an animation on an AnimationState track. References to a track entry must not be kept after the dispose event occurs.
- TrackEntry Properties
- alpha: float
- Values < 1 mix this animation with the skeleton's current pose (usually the pose resulting from lower tracks). Defaults to 1, which overwrites the skeleton's current pose with this animation. Typically track 0 is used to completely pose the skeleton, then alpha is used on higher tracks. It doesn't make sense to use alpha on track 0 if the skeleton pose is from the last frame render.
- alphaAttachmentThreshold: float
- When alpha is greater than
alphaAttachmentThreshold
, attachment timelines are applied. Defaults to 0, so attachment timelines are always applied. - animation: Animation
- The animation to apply for this track entry.
- animationEnd: float
- Seconds for the last frame of this animation. Non-looping animations won't play past this time. Looping animations will loop back to animationStart at this time. Defaults to the animation duration.
- animationLast: float
- The time in seconds this animation was last applied. Some timelines use this for one-time triggers. Eg, when this animation is applied, event timelines will fire all events between the
animationLast
time (exclusive) andanimationTime
(inclusive). Defaults to -1 to ensure triggers on frame 0 happen the first time this animation is applied. - animationStart: float
- Seconds when this animation starts, both initially and after looping. Defaults to 0. When changing the
animationStart
time, it often makes sense to set animationLast to the same value to prevent timeline keys before the start time from triggering. - animationTime: float readonly
- Uses trackTime to compute the
animationTime
. When thetrackTime
is 0, theanimationTime
is equal to theanimationStart
time. TheanimationTime
is between animationStart and animationEnd, except if this track entry is non-looping and animationEnd is >= to the animation duration, thenanimationTime
continues to increase past animationEnd. - delay: float
- Seconds to postpone playing the animation. When this track entry is the current track entry,
delay
postpones incrementing the trackTime. When this track entry is queued,delay
is the time from the start of the previous animation to when this track entry will become the current track entry (ie when the previous track entry trackTime >= this track entry'sdelay
). timeScale affects the delay. When using addAnimation with adelay
<= 0, the delay is set using the mix duration from the AnimationStateData. If mixDuration is set afterward, the delay may need to be adjusted. - eventThreshold: float
- When the mix percentage (mixTime / mixDuration) is less than the
eventThreshold
, event timelines are applied while this animation is being mixed out. Defaults to 0, so event timelines are not applied while this animation is being mixed out. - holdPrevious: bool
- If true, when mixing from the previous animation to this animation, the previous animation is applied as normal instead of being mixed out. When mixing between animations that key the same property, if a lower track also keys that property then the value will briefly dip toward the lower track value during the mix. This happens because the first animation mixes from 100% to 0% while the second animation mixes from 0% to 100%. Setting
holdPrevious
to true applies the first animation at 100% during the mix so the lower track value is overwritten. Such dipping does not occur on the lowest track which keys the property, only when a higher track also keys the property. Snapping will occur ifholdPrevious
is true and this animation does not key all the same properties as the previous animation. - listener: AnimationStateListener
- The listener for events generated by this track entry, or null. A track entry returned from setAnimation is already the current animation for the track, so the track entry listener start will not be called.
- loop: bool
- If true, the animation will repeat. If false it will not, instead its last frame is applied if played beyond its duration.
- mixAttachmentThreshold: float
- When the mix percentage (mixTime / mixDuration) is less than the
mixAttachmentThreshold
, attachment timelines are applied while this animation is being mixed out. Defaults to 0, so attachment timelines are not applied while this animation is being mixed out. - mixBlend: MixBlend
- Controls how properties keyed in the animation are mixed with lower tracks. Defaults to replace. Track entries on track 0 ignore this setting and always use first. The
mixBlend
can be set for a new track entry only before apply is next called. - mixDrawOrderThreshold: float
- When the mix percentage (mixTime / mixDuration) is less than the
mixDrawOrderThreshold
, draw order timelines are applied while this animation is being mixed out. Defaults to 0, so draw order timelines are not applied while this animation is being mixed out. - mixDuration: float
- Seconds for mixing from the previous animation to this animation. Defaults to the value provided by AnimationStateData getMix based on the animation before this animation (if any). A mix duration of 0 still mixes out over one frame to provide the track entry being mixed out a chance to revert the properties it was animating. A mix duration of 0 can be set at any time to end the mix on the next update. The
mixDuration
can be set manually rather than use the value from getMix. In that case, themixDuration
can be set for a new track entry only before update is next called. When using addAnimation with adelay
<= 0, the delay is set using the mix duration from the AnimationStateData. IfmixDuration
is set afterward, the delay may need to be adjusted. For example:
entry.delay = entry.previous.getTrackComplete() - entry.mixDuration;
Alternatively, setMixDuration can be used to recompute the delay:
entry.setMixDuration(0.25f, 0);
- mixTime: float
- Seconds from 0 to the mixDuration when mixing from the previous animation to this animation. May be slightly more than
mixDuration
when the mix is complete. - mixingFrom: TrackEntry readonly
- The track entry for the previous animation when mixing from the previous animation to this animation, or null if no mixing is currently occurring. When mixing from multiple animations,
mixingFrom
makes up a linked list. - mixingTo: TrackEntry readonly
- The track entry for the next animation when mixing from this animation to the next animation, or null if no mixing is currently occurring. When mixing to multiple animations,
mixingTo
makes up a linked list. - next: TrackEntry readonly
- The animation queued to start after this animation, or null if there is none.
next
makes up a doubly linked list. See clearNext to truncate the list. - previous: TrackEntry readonly
- The animation queued to play before this animation, or null.
previous
makes up a doubly linked list. - reverse: bool
- If true, the animation will be applied in reverse. Events are not fired when an animation is applied in reverse.
- shortestRotation: bool
- If true, mixing rotation between tracks always uses the shortest rotation direction. If the rotation is animated, the shortest rotation direction may change during the mix. If false, the shortest rotation direction is remembered when the mix starts and the same direction is used for the rest of the mix. Defaults to false.
- timeScale: float
- Multiplier for the delta time when this track entry is updated, causing time for this animation to pass slower or faster. Defaults to 1. Values < 0 are not supported. To play an animation in reverse, use reverse. mixTime is not affected by track entry time scale, so mixDuration may need to be adjusted to match the animation speed. When using addAnimation with a
delay
<= 0, the delay is set using the mix duration from the AnimationStateData, assuming time scale to be 1. If the time scale is not 1, the delay may need to be adjusted. See AnimationState timeScale for affecting all animations. - trackComplete: float readonly
- If this track entry is non-looping, the track time in seconds when animationEnd is reached, or the current trackTime if it has already been reached. If this track entry is looping, the track time when this animation will reach its next animationEnd (the next loop completion).
- trackEnd: float
- The track time in seconds when this animation will be removed from the track. Defaults to the highest possible float value, meaning the animation will be applied until a new animation is set or the track is cleared. If the track end time is reached, no other animations are queued for playback, and mixing from any previous animations is complete, then the properties keyed by the animation are set to the setup pose and the track is cleared. It may be desired to use addEmptyAnimation rather than have the animation abruptly cease being applied.
- trackIndex: int readonly
- The index of the track where this track entry is either current or queued. See getCurrent.
- trackTime: float
- Current time in seconds this track entry has been the current track entry. The track time determines animationTime. The track time can be set to start the animation at a time other than 0, without affecting looping.
- TrackEntry Methods
isComplete ( ): bool - Returns true if at least one loop has been completed. See complete.
isEmptyAnimation ( ): bool - Returns true if this entry is for the empty animation. See setEmptyAnimation, addEmptyAnimation, and setEmptyAnimations.
isNextReady ( ): bool - Returns true if there is a next track entry and it will become the current track entry during the next update.
resetRotationDirections ( ): void - Resets the rotation directions for mixing this entry's rotate timelines. This can be useful to avoid bones rotating the long way around when using alpha and starting animations on other tracks. Mixing with replace involves finding a rotation between two others, which has two possible solutions: the short way or the long way around. The two rotations likely change over time, so which direction is the short or long way also changes. If the short way was always chosen, bones would flip to the other side when that direction became the long way. TrackEntry chooses the short way the first time it is applied and remembers that direction.
setMixDuration ( float mixDuration, float delay): void - Sets both mixDuration and delay.
delay
If > 0, sets delay. If <= 0, the delay set is the duration of the previous track entry minus the specified mix duration plus the specifieddelay
(ie the mix ends at (delay
= 0) or before (delay
< 0) the previous track entry duration). If the previous entry is looping, its next loop completion is used instead of its duration.
wasApplied ( ): bool - Returns true if this track entry has been applied at least once. See apply.
Stores information about texture regions in one or more texture pages.Creating an atlas takes the atlas data file and a TextureLoader.
- Atlas Properties
- pages: list<AtlasPage>
- An atlas page for each texture.
- regions: list<AtlasRegion>
- The atlas regions across all pages.
- Atlas Methods
dispose ( ): void - Uses the TextureLoader to unload each AtlasPage rendererObject.
findRegion ( string name): AtlasRegion - Returns the first region found with the specified name, or null if it was not found. String comparison is used to find the region so the result should be cached rather than calling this method multiple times.
Settings for an atlas backing texture.
- AtlasPage Properties
- format: Format
- The memory format to use for the texture.
- height: int
- The height in pixels of the image file.
- magFilter: TextureFilter
- The texture's magnification filter.
- minFilter: TextureFilter
- The texture's minification filter.
- name: string
- The name of the image file for the texture.
- rendererObject: object
- A game toolkit specific object used by rendering code, usually set by a TextureLoader.See Loading skeleton data.
- uWrap: TextureWrap
- The X axis texture wrap setting.
- vWrap: TextureWrap
- The Y axis texture wrap setting.
- width: int
- The width in pixels of the image file.
A texture region on an atlas page.
- AtlasRegion Properties
- height: int
- The height in pixels of the unrotated texture region after whitepace stripping.
- index: int
- The number at the end of the original image file name, or -1 if none.When sprites are packed, if the original file name ends with a number, it is stored as the index and is not considered as part of the region's name. This is useful for keeping animation frames in order.
- name: string
- The name of the region.
- offsetX: float
- Pixels stripped from the left of the unrotated texture region.
- offsetY: float
- Pixels stripped from the bottom of the unrotated texture region.
- originalHeight: int
- The height in pixels of the unrotated texture region before whitespace stripping.
- originalWidth: int
- The width in pixels of the unrotated texture region before whitespace stripping.
- pads: int[]
- The ninepatch padding, or null if not a ninepatch or the ninepatch has no padding. Has 4 entries: left, right, top, bottom.
- page: AtlasPage
- The atlas page this region belongs to.
- rotate: bool
- If true, the texture region is stored in the atlas rotated 90 degrees counterclockwise.
- splits: int[]
- The ninepatch splits, or null if not a ninepatch. Has 4 entries: left, right, top, bottom.
- u: float
- The normalized (0-1) texture coordinate of the left edge of the texture region.
- u2: float
- The normalized (0-1) texture coordinate of the right edge of the texture region.
- v: float
- The normalized (0-1) texture coordinate of the top edge of the texture region.
- v2: float
- The normalized (0-1) texture coordinate of the bottom edge of the texture region.
- width: int
- The width in pixels of the unrotated texture region after whitespace stripping.
- x: int
- Pixels from the left edge of the texture to the left edge of the texture region.
- y: int
- Pixels from the bottom edge of the texture to the bottom edge of the texture region.
The memory format to use when loading an image into the AtlasPage texture.
The filtering for magnification or minification of the AtlasPage texture.
- TextureFilter Values
- Nearest
- Linear
- MipMap
- MipMapNearestNearest
- MipMapLinearNearest
- MipMapNearestLinear
- MipMapLinearLinear
The interface which can be implemented to customize loading of AtlasPage images for an Atlas.See Loading skeleton data.
- TextureLoader Methods
load ( AtlasPage page, string path): void - Loads a texture using the AtlasPage name or the image at the specified path and sets the AtlasPage rendererObject.
unload ( object rendererObject): void - Unloads the
rendererObject
previously loaded in load.
The texture wrapping mode for UVs outside the AtlasPage texture.
The base class for all attachments.
- Attachment Properties
- name: string readonly
- The attachment's name.
- Attachment Methods
copy ( ): Attachment - Returns a copy of the attachment.
An attachment with vertices that make up a polygon. Can be used for hit detection, creating physics bodies, spawning particle effects, and more. See SkeletonBounds and Bounding Boxes in the Spine User Guide.
- BoundingBoxAttachment Properties
- bones: int[] from VertexAttachment
- The bones which affect the vertices. The array entries are, for each vertex, the number of bones affecting the vertex followed by that many bone indices, which is the index of the bone in bones. Will be null if this attachment has no weights.
- color: Color
- The color of the bounding box as it was in Spine, or a default color if nonessential data was not exported. Bounding boxes are not usually rendered at runtime.
- id: int readonly, from VertexAttachment
- Returns a unique ID for this attachment.
- name: string readonly, from Attachment
- The attachment's name.
- timelineAttachment: Attachment from VertexAttachment
- Timelines for the timeline attachment are also applied to this attachment. May be null if no attachment-specific timelines should be applied.
- vertices: float[] from VertexAttachment
- The vertex positions in the bone's coordinate system. For a non-weighted attachment, the values are
x,y
entries for each vertex. For a weighted attachment, the values arex,y,weight
entries for each bone affecting each vertex. - worldVerticesLength: int from VertexAttachment
- The maximum number of world vertex values that can be output by computeWorldVertices using the
count
parameter.
- BoundingBoxAttachment Methods
computeWorldVertices ( Slot slot, int start, int count, float[] worldVertices, int offset, int stride): void from VertexAttachment - Transforms the attachment's local vertices to world coordinates. If the slot's deform is not empty, it is used to deform the vertices. See World transforms in the Spine Runtimes Guide.See VertexAttachment computeWorldVertices.
copy ( ): BoundingBoxAttachment from Attachment - Returns a copy of the attachment.
ClippingAttachment
extends VertexAttachmentAn attachment with vertices that make up a polygon used for clipping the rendering of other attachments.
- ClippingAttachment Properties
- bones: int[] from VertexAttachment
- The bones which affect the vertices. The array entries are, for each vertex, the number of bones affecting the vertex followed by that many bone indices, which is the index of the bone in bones. Will be null if this attachment has no weights.
- color: Color
- The color of the clipping attachment as it was in Spine, or a default color if nonessential data was not exported. Clipping attachments are not usually rendered at runtime.
- endSlot: SlotData
- Clipping is performed between the clipping attachment's slot and the end slot. If null clipping is done until the end of the skeleton's rendering.
- id: int readonly, from VertexAttachment
- Returns a unique ID for this attachment.
- name: string readonly, from Attachment
- The attachment's name.
- timelineAttachment: Attachment from VertexAttachment
- Timelines for the timeline attachment are also applied to this attachment. May be null if no attachment-specific timelines should be applied.
- vertices: float[] from VertexAttachment
- The vertex positions in the bone's coordinate system. For a non-weighted attachment, the values are
x,y
entries for each vertex. For a weighted attachment, the values arex,y,weight
entries for each bone affecting each vertex. - worldVerticesLength: int from VertexAttachment
- The maximum number of world vertex values that can be output by computeWorldVertices using the
count
parameter.
- ClippingAttachment Methods
computeWorldVertices ( Slot slot, int start, int count, float[] worldVertices, int offset, int stride): void from VertexAttachment - Transforms the attachment's local vertices to world coordinates. If the slot's deform is not empty, it is used to deform the vertices. See World transforms in the Spine Runtimes Guide.See VertexAttachment computeWorldVertices.
copy ( ): ClippingAttachment from Attachment - Returns a copy of the attachment.
- HasTextureRegion Properties
- color: Color
- The color to tint the attachment.
- path: string
- The name used to find the region.
- region: TextureRegion
- sequence: Sequence
- HasTextureRegion Methods
updateRegion ( ): void - Updates any values the attachment calculates using the region. Must be called after setting the region or if the region's properties are changed.
An attachment that displays a textured mesh. A mesh has hull vertices and internal vertices within the hull. Holes are not supported. Each vertex has UVs (texture coordinates) and triangles are used to map an image on to the mesh. See Mesh attachments in the Spine User Guide.
- MeshAttachment Properties
- bones: int[] from VertexAttachment
- The bones which affect the vertices. The array entries are, for each vertex, the number of bones affecting the vertex followed by that many bone indices, which is the index of the bone in bones. Will be null if this attachment has no weights.
- color: Color
- edges: int[]
- Vertex index pairs describing edges for controlling triangulation, or be null if nonessential data was not exported. Mesh triangles will never cross edges. Triangulation is not performed at runtime.
- height: float
- The height of the mesh's image, or zero if nonessential data was not exported.
- hullLength: int
- The number of entries at the beginning of vertices that make up the mesh hull.
- id: int readonly, from VertexAttachment
- Returns a unique ID for this attachment.
- name: string readonly, from Attachment
- The attachment's name.
- parentMesh: MeshAttachment
- The parent mesh if this is a linked mesh, else null. A linked mesh shares the bones, vertices, regionUVs, triangles, hullLength, edges, width, and height with the parent mesh, but may have a different name or path (and therefore a different texture).
- path: string
- regionHeight: float
- The height in pixels of the unrotated texture region after whitepace stripping.
- regionOffsetX: float
- Pixels stripped from the left of the unrotated texture region.
- regionOffsetY: float
- Pixels stripped from the bottom of the unrotated texture region.
- regionOriginalHeight: float
- The height in pixels of the unrotated texture region before whitespace stripping.
- regionOriginalWidth: float
- The width in pixels of the unrotated texture region before whitespace stripping.
- regionRotate: bool
- If true, the texture region is stored in the atlas rotated 90 degrees counterclockwise.
- regionU: float
- The normalized (0-1) texture coordinate of the left edge of the texture region.
- regionU2: float
- The normalized (0-1) texture coordinate of the right edge of the texture region.
- regionUVs: float[]
- The UV pair for each vertex, normalized within the texture region.
- regionV: float
- The normalized (0-1) texture coordinate of the top edge of the texture region.
- regionV2: float
- The normalized (0-1) texture coordinate of the bottom edge of the texture region.
- regionWidth: float
- The width in pixels of the unrotated texture region after whitespace stripping.
- rendererObject: object
- A game toolkit specific object used by rendering code, usually set by an AttachmentLoader.See Generic rendering
- sequence: Sequence
- timelineAttachment: Attachment from VertexAttachment
- Timelines for the timeline attachment are also applied to this attachment. May be null if no attachment-specific timelines should be applied.
- triangles: int[]
- Triplets of vertex indices which describe the mesh's triangulation.
- uvs: float[]
- The UV pair for each vertex, normalized within the entire texture. See updateRegion.
- vertices: float[] from VertexAttachment
- The vertex positions in the bone's coordinate system. For a non-weighted attachment, the values are
x,y
entries for each vertex. For a weighted attachment, the values arex,y,weight
entries for each bone affecting each vertex. - width: float
- The width of the mesh's image, or zero if nonessential data was not exported.
- worldVerticesLength: int from VertexAttachment
- The maximum number of world vertex values that can be output by computeWorldVertices using the
count
parameter.
- MeshAttachment Methods
computeWorldVertices ( Slot slot, int start, int count, float[] worldVertices, int offset, int stride): void from VertexAttachment - If the attachment has a sequence, the region may be changed.See VertexAttachment computeWorldVertices.
copy ( ): MeshAttachment from Attachment - Returns a copy of the attachment.
newLinkedMesh ( ): MeshAttachment - Returns a new mesh with the parentMesh set to this mesh's parent mesh, if any, else to this mesh.
updateRegion ( ): void - Calculates uvs using the regionUVs and region. Must be called if the region, the region's properties, or the regionUVs are changed.
updateUVs ( ): void - Calculates the uvs using the regionUVs and other region settings. Must be called after changing region settings.
PathAttachment
extends VertexAttachmentAn attachment whose vertices make up a composite Bezier curve. See PathConstraint and Paths in the Spine User Guide.
- PathAttachment Properties
- bones: int[] from VertexAttachment
- The bones which affect the vertices. The array entries are, for each vertex, the number of bones affecting the vertex followed by that many bone indices, which is the index of the bone in bones. Will be null if this attachment has no weights.
- closed: bool
- If true, the start and end knots are connected.
- color: Color
- The color of the path as it was in Spine, or a default color if nonessential data was not exported. Paths are not usually rendered at runtime.
- constantSpeed: bool
- If true, additional calculations are performed to make computing positions along the path more accurate and movement along the path have a constant speed.
- id: int readonly, from VertexAttachment
- Returns a unique ID for this attachment.
- lengths: float[]
- The lengths along the path in the setup pose from the start of the path to the end of each Bezier curve.
- name: string readonly, from Attachment
- The attachment's name.
- timelineAttachment: Attachment from VertexAttachment
- Timelines for the timeline attachment are also applied to this attachment. May be null if no attachment-specific timelines should be applied.
- vertices: float[] from VertexAttachment
- The vertex positions in the bone's coordinate system. For a non-weighted attachment, the values are
x,y
entries for each vertex. For a weighted attachment, the values arex,y,weight
entries for each bone affecting each vertex. - worldVerticesLength: int from VertexAttachment
- The maximum number of world vertex values that can be output by computeWorldVertices using the
count
parameter.
- PathAttachment Methods
computeWorldVertices ( Slot slot, int start, int count, float[] worldVertices, int offset, int stride): void from VertexAttachment - Transforms the attachment's local vertices to world coordinates. If the slot's deform is not empty, it is used to deform the vertices. See World transforms in the Spine Runtimes Guide.See VertexAttachment computeWorldVertices.
copy ( ): PathAttachment from Attachment - Returns a copy of the attachment.
PointAttachment
extends AttachmentAn attachment which is a single point and a rotation. This can be used to spawn projectiles, particles, etc. A bone can be used in similar ways, but a PointAttachment is slightly less expensive to compute and can be hidden, shown, and placed in a skin. See Point Attachments in the Spine User Guide.
- PointAttachment Properties
- color: Color
- The color of the point attachment as it was in Spine, or a default clor if nonessential data was not exported. Point attachments are not usually rendered at runtime.
- name: string readonly, from Attachment
- The attachment's name.
- rotation: float
- x: float
- y: float
- PointAttachment Methods
computeWorldPosition ( Bone bone, 2-tuple point): 2-tuple computeWorldRotation ( Bone bone): float copy ( ): PointAttachment from Attachment - Returns a copy of the attachment.
An attachment that displays a textured quadrilateral. See Region attachments in the Spine User Guide.
- RegionAttachment Properties
- color: Color
- height: float
- The height of the region attachment in Spine.
- name: string readonly, from Attachment
- The attachment's name.
- offset: float[] readonly
- For each of the 4 vertices, a pair of
x,y
values that is the local position of the vertex. See updateRegion. - path: string
- regionHeight: float
- The height in pixels of the unrotated texture region after whitepace stripping.
- regionOffsetX: float
- Pixels stripped from the left of the unrotated texture region.
- regionOffsetY: float
- Pixels stripped from the bottom of the unrotated texture region.
- regionOriginalHeight: float
- The height in pixels of the unrotated texture region before whitespace stripping.
- regionOriginalWidth: float
- The width in pixels of the unrotated texture region before whitespace stripping.
- regionWidth: float
- The width in pixels of the unrotated texture region after whitespace stripping.
- rendererObject: object
- A game toolkit specific object used by rendering code, usually set by an AttachmentLoader.See Generic rendering
- rotation: float
- The local rotation.
- scaleX: float
- The local scaleX.
- scaleY: float
- The local scaleY.
- sequence: Sequence
- uvs: float[] readonly
- width: float
- The width of the region attachment in Spine.
- x: float
- The local x translation.
- y: float
- The local y translation.
- RegionAttachment Methods
computeWorldVertices ( Slot slot, float[] worldVertices, int offset, int stride): void - Transforms the attachment's four vertices to world coordinates. If the attachment has a sequence, the region may be changed. See World transforms in the Spine Runtimes Guide.
worldVertices
The output world vertices. Must have a length >=offset
+ 8.offset
TheworldVertices
index to begin writing values.stride
The number ofworldVertices
entries between the value pairs written.
computeWorldVertices ( Bone bone, float[] worldVertices): void copy ( ): RegionAttachment from Attachment - Returns a copy of the attachment.
setUVs ( float u, float v, float u2, float v2, bool rotate): void - Sets the normalized (0-1) texture coordinates of the left, top, right, and bottom edges of the texture region.
u
The left edge.v
The top edge.u2
The right edge.v2
The bottom edge.rotate
If true, the texture region is stored in the atlas rotated 90 degrees counterclockwise.
updateRegion ( ): void - Calculates the offset and uvs using the region and the attachment's transform. Must be called if the region, the region's properties, or the transform are changed.
VertexAttachment
extends AttachmentBase class for an attachment with vertices that are transformed by one or more bones and can be deformed by a slot's deform.
- VertexAttachment Properties
- bones: int[]
- The bones which affect the vertices. The array entries are, for each vertex, the number of bones affecting the vertex followed by that many bone indices, which is the index of the bone in bones. Will be null if this attachment has no weights.
- id: int readonly
- Returns a unique ID for this attachment.
- name: string readonly, from Attachment
- The attachment's name.
- timelineAttachment: Attachment
- Timelines for the timeline attachment are also applied to this attachment. May be null if no attachment-specific timelines should be applied.
- vertices: float[]
- The vertex positions in the bone's coordinate system. For a non-weighted attachment, the values are
x,y
entries for each vertex. For a weighted attachment, the values arex,y,weight
entries for each bone affecting each vertex. - worldVerticesLength: int
- The maximum number of world vertex values that can be output by computeWorldVertices using the
count
parameter.
- VertexAttachment Methods
computeWorldVertices ( Slot slot, int start, int count, float[] worldVertices, int offset, int stride): void - Transforms the attachment's local vertices to world coordinates. If the slot's deform is not empty, it is used to deform the vertices. See World transforms in the Spine Runtimes Guide.
start
The index of the first vertices value to transform. Each vertex has 2 values, x and y.count
The number of world vertex values to output. Must be <= worldVerticesLength -start
.worldVertices
The output world vertices. Must have a length >=offset
+count
*stride
/ 2.offset
TheworldVertices
index to begin writing values.stride
The number ofworldVertices
entries between the value pairs written.
copy ( ): Attachment from Attachment - Returns a copy of the attachment.
The interface which can be implemented to customize creating and populating attachments. See Loading skeleton data in the Spine Runtimes Guide.
- AttachmentLoader Methods
newBoundingBoxAttachment ( Skin skin, string name): BoundingBoxAttachment <return>
May be null to not load the attachment.
newClippingAttachment ( Skin skin, string name): ClippingAttachment <return>
May be null to not load the attachment.
newMeshAttachment ( Skin skin, string name, string path, Sequence sequence): MeshAttachment sequence
May be null.<return>
May be null to not load the attachment. In that case null should also be returned for child meshes.
newPathAttachment ( Skin skin, string name): PathAttachment <return>
May be null to not load the attachment.
newPointAttachment ( Skin skin, string name): PointAttachment <return>
May be null to not load the attachment.
newRegionAttachment ( Skin skin, string name, string path, Sequence sequence): RegionAttachment sequence
May be null.<return>
May be null to not load the attachment.
AtlasAttachmentLoader
implements AttachmentLoaderAn AttachmentLoader that configures attachments using texture regions from an Atlas. See Loading skeleton data in the Spine Runtimes Guide.
- AtlasAttachmentLoader Methods
newBoundingBoxAttachment ( Skin skin, string name): BoundingBoxAttachment from AttachmentLoader newClippingAttachment ( Skin skin, string name): ClippingAttachment from AttachmentLoader newMeshAttachment ( Skin skin, string name, string path, Sequence sequence): MeshAttachment from AttachmentLoader sequence
May be null.
newPathAttachment ( Skin skin, string name): PathAttachment from AttachmentLoader newPointAttachment ( Skin skin, string name): PointAttachment from AttachmentLoader newRegionAttachment ( Skin skin, string name, string path, Sequence sequence): RegionAttachment from AttachmentLoader sequence
May be null.
Stores the setup pose for a Bone.
- BoneData Properties
- color: Color
- The color of the bone as it was in Spine, or a default color if nonessential data was not exported. Bones are not usually rendered at runtime.
- icon: string
- The bone icon as it was in Spine, or null if nonessential data was not exported.
- index: int readonly
- The index of the bone in bones.
- inherit: Inherit
- Determines how parent world transforms affect this bone.
- length: float
- The bone's length.
- name: string readonly
- The name of the bone, which is unique across all bones in the skeleton.
- parent: BoneData readonly
- rotation: float
- The local rotation in degrees, counter clockwise.
- scaleX: float
- The local scaleX.
- scaleY: float
- The local scaleY.
- shearX: float
- The local shearX.
- shearY: float
- The local shearX.
- skinRequired: bool
- When true, updateWorldTransform only updates this bone if the skin contains this bone. See bones.
- visible: bool
- False if the bone was hidden in Spine and nonessential data was exported. Does not affect runtime rendering.
- x: float
- The local x translation.
- y: float
- The local y translation.
Stores a bone's current pose. A bone has a local transform which is used to compute its world transform. A bone also has an applied transform, which is a local transform that can be applied to compute the world transform. The local transform and applied transform may differ if a constraint or application code modifies the world transform after it was computed from the local transform.
- Bone Properties
- a: float
- Part of the world transform matrix for the X axis. If changed, updateAppliedTransform should be called.
- aRotation: float
- The applied local rotation in degrees, counter clockwise.
- aScaleX: float
- The applied local scaleX.
- aScaleY: float
- The applied local scaleY.
- aShearX: float
- The applied local shearX.
- aShearY: float
- The applied local shearY.
- aX: float
- The applied local x translation.
- aY: float
- The applied local y translation.
- b: float
- Part of the world transform matrix for the Y axis. If changed, updateAppliedTransform should be called.
- c: float
- Part of the world transform matrix for the X axis. If changed, updateAppliedTransform should be called.
- children: list<Bone> readonly
- The immediate children of this bone.
- d: float
- Part of the world transform matrix for the Y axis. If changed, updateAppliedTransform should be called.
- data: BoneData readonly
- The bone's setup pose data.
- inherit: Inherit
- Determines how parent world transforms affect this bone.
- parent: Bone readonly
- The parent bone, or null if this is the root bone.
- rotation: float
- The local rotation in degrees, counter clockwise.
- scaleX: float
- The local scaleX.
- scaleY: float
- The local scaleY.
- shearX: float
- The local shearX.
- shearY: float
- The local shearY.
- skeleton: Skeleton readonly
- The skeleton this bone belongs to.
- worldRotationX: float readonly
- The world rotation for the X axis, calculated using a and c.
- worldRotationY: float readonly
- The world rotation for the Y axis, calculated using b and d.
- worldScaleX: float readonly
- The magnitude (always positive) of the world scale X, calculated using a and c.
- worldScaleY: float readonly
- The magnitude (always positive) of the world scale Y, calculated using b and d.
- worldX: float
- The world X position. If changed, updateAppliedTransform should be called.
- worldY: float
- The world Y position. If changed, updateAppliedTransform should be called.
- x: float
- The local x translation.
- y: float
- The local y translation.
- Bone Methods
isActive ( ): bool localToWorld ( 2-tuple local): 2-tuple - Transforms a point from the bone's local coordinates to world coordinates.
localToWorldRotation ( float localRotation): float - Transforms a local rotation to a world rotation.
parentToWorld ( 2-tuple world): 2-tuple - Transforms a point from the parent bone's coordinates to world coordinates.
rotateWorld ( float degrees): void - Rotates the world transform the specified amount. After changes are made to the world transform, updateAppliedTransform should be called and update will need to be called on any child bones, recursively.
setToSetupPose ( ): void - Sets this bone's local transform to the setup pose.
update ( Physics physics): void - Computes the world transform using the parent bone and this bone's local applied transform.
updateAppliedTransform ( ): void - Computes the applied transform values from the world transform. If the world transform is modified (by a constraint, rotateWorld, etc) then this method should be called so the applied transform matches the world transform. The applied transform may be needed by other code (eg to apply another constraint). Some information is ambiguous in the world transform, such as -1,-1 scale versus 180 rotation. The applied transform after calling this method is equivalent to the local transform used to compute the world transform, but may not be identical.
updateWorldTransform ( float x, float y, float rotation, float scaleX, float scaleY, float shearX, float shearY): void - Computes the world transform using the parent bone and the specified local transform. The applied transform is set to the specified local transform. Child bones are not updated. See World transforms in the Spine Runtimes Guide.
updateWorldTransform ( ): void - Computes the world transform using the parent bone and this bone's local transform. See updateWorldTransform.
worldToLocal ( 2-tuple world): 2-tuple - Transforms a point from world coordinates to the bone's local coordinates.
worldToLocalRotation ( float worldRotation): float - Transforms a world rotation to a local rotation.
worldToParent ( 2-tuple world): 2-tuple - Transforms a point from world coordinates to the parent bone's local coordinates.
Determines how a bone inherits world transforms from parent bones.
The base class for all constraint datas.
- ConstraintData Properties
- name: string readonly
- The constraint's name, which is unique across all constraints in the skeleton of the same type.
- order: int
- The ordinal of this constraint for the order a skeleton's constraints will be applied by updateWorldTransform.
- skinRequired: bool
- When true, updateWorldTransform only updates this constraint if the skin contains this constraint. See constraints.
IkConstraintData
extends ConstraintDataStores the setup pose for an IkConstraint. See IK constraints in the Spine User Guide.
- IkConstraintData Properties
- bendDirection: int
- For two bone IK, controls the bend direction of the IK bones, either 1 or -1.
- bones: list<BoneData> readonly
- The bones that are constrained by this IK constraint.
- compress: bool
- For one bone IK, when true and the target is too close, the bone is scaled to reach it.
- mix: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained rotation. For two bone IK: if the parent bone has local nonuniform scale, the child bone's local Y translation is set to 0.
- name: string readonly, from ConstraintData
- The constraint's name, which is unique across all constraints in the skeleton of the same type.
- order: int from ConstraintData
- The ordinal of this constraint for the order a skeleton's constraints will be applied by updateWorldTransform.
- skinRequired: bool from ConstraintData
- When true, updateWorldTransform only updates this constraint if the skin contains this constraint. See constraints.
- softness: float
- For two bone IK, the target bone's distance from the maximum reach of the bones where rotation begins to slow. The bones will not straighten completely until the target is this far out of range.
- stretch: bool
- When true and the target is out of range, the parent bone is scaled to reach it. For two bone IK: 1) the child bone's local Y translation is set to 0, 2) stretch is not applied if softness is > 0, and 3) if the parent bone has local nonuniform scale, stretch is not applied.
- target: BoneData
- The bone that is the IK target.
- uniform: bool
- When true and compress or stretch is used, the bone is scaled on both the X and Y axes.
Stores the current pose for an IK constraint. An IK constraint adjusts the rotation of 1 or 2 constrained bones so the tip of the last bone is as close to the target bone as possible. See IK constraints in the Spine User Guide.
- IkConstraint Properties
- bendDirection: int
- For two bone IK, controls the bend direction of the IK bones, either 1 or -1.
- bones: list<Bone> readonly
- The bones that will be modified by this IK constraint.
- compress: bool
- For one bone IK, when true and the target is too close, the bone is scaled to reach it.
- data: IkConstraintData readonly
- The IK constraint's setup pose data.
- mix: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained rotation. For two bone IK: if the parent bone has local nonuniform scale, the child bone's local Y translation is set to 0.
- softness: float
- For two bone IK, the target bone's distance from the maximum reach of the bones where rotation begins to slow. The bones will not straighten completely until the target is this far out of range.
- stretch: bool
- When true and the target is out of range, the parent bone is scaled to reach it. For two bone IK: 1) the child bone's local Y translation is set to 0, 2) stretch is not applied if softness is > 0, and 3) if the parent bone has local nonuniform scale, stretch is not applied.
- target: Bone
- The bone that is the IK target.
- IkConstraint Methods
isActive ( ): bool setToSetupPose ( ): void update ( Physics physics): void - Applies the constraint to the constrained bones.
apply ( Bone parent, Bone child, float targetX, float targetY, int bendDir, bool stretch, bool uniform, float softness, float alpha): void static - Applies 2 bone IK. The target is specified in the world coordinate system.
child
A direct descendant of the parent bone.
apply ( Bone bone, float targetX, float targetY, bool compress, bool stretch, bool uniform, float alpha): void static - Applies 1 bone IK. The target is specified in the world coordinate system.
PathConstraintData
extends ConstraintDataStores the setup pose for a PathConstraint. See Path constraints in the Spine User Guide.
- PathConstraintData Properties
- bones: list<BoneData> readonly
- The bones that will be modified by this path constraint.
- mixRotate: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained rotation.
- mixX: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained translation X.
- mixY: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained translation Y.
- name: string readonly, from ConstraintData
- The constraint's name, which is unique across all constraints in the skeleton of the same type.
- offsetRotation: float
- An offset added to the constrained bone rotation.
- order: int from ConstraintData
- The ordinal of this constraint for the order a skeleton's constraints will be applied by updateWorldTransform.
- position: float
- The position along the path.
- positionMode: PositionMode
- The mode for positioning the first bone on the path.
- rotateMode: RotateMode
- The mode for adjusting the rotation of the bones.
- skinRequired: bool from ConstraintData
- When true, updateWorldTransform only updates this constraint if the skin contains this constraint. See constraints.
- spacing: float
- The spacing between bones.
- spacingMode: SpacingMode
- The mode for positioning the bones after the first bone on the path.
- target: SlotData
- The slot whose path attachment will be used to constrained the bones.
Stores the current pose for a path constraint. A path constraint adjusts the rotation, translation, and scale of the constrained bones so they follow a PathAttachment. See Path constraints in the Spine User Guide.
- PathConstraint Properties
- bones: list<Bone> readonly
- The bones that will be modified by this path constraint.
- data: PathConstraintData readonly
- The path constraint's setup pose data.
- mixRotate: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained rotation.
- mixX: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained translation X.
- mixY: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained translation Y.
- position: float
- The position along the path.
- spacing: float
- The spacing between bones.
- target: Slot
- The slot whose path attachment will be used to constrained the bones.
- PathConstraint Methods
isActive ( ): bool setToSetupPose ( ): void update ( Physics physics): void - Applies the constraint to the constrained bones.
Controls how the first bone is positioned along the path. See Position mode in the Spine User Guide.
Controls how bones are rotated, translated, and scaled to match the path. See Rotate mode in the Spine User Guide.
- RotateMode Values
- tangent
- chain
- chainScale
- When chain scale, constrained bones should all have the same parent. That way when the path constraint scales a bone, it doesn't affect other constrained bones.
Controls how bones after the first bone are positioned along the path. See Spacing mode in the Spine User Guide.
PhysicsConstraintData
extends ConstraintDataStores the setup pose for a PhysicsConstraint. See Physics constraints in the Spine User Guide.
- PhysicsConstraintData Properties
- bone: BoneData
- The bone constrained by this physics constraint.
- damping: float
- dampingGlobal: bool
- gravity: float
- gravityGlobal: bool
- inertia: float
- inertiaGlobal: bool
- limit: float
- massGlobal: bool
- massInverse: float
- mix: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained poses.
- mixGlobal: bool
- name: string readonly, from ConstraintData
- The constraint's name, which is unique across all constraints in the skeleton of the same type.
- order: int from ConstraintData
- The ordinal of this constraint for the order a skeleton's constraints will be applied by updateWorldTransform.
- rotate: float
- scaleX: float
- shearX: float
- skinRequired: bool from ConstraintData
- When true, updateWorldTransform only updates this constraint if the skin contains this constraint. See constraints.
- step: float
- strength: float
- strengthGlobal: bool
- wind: float
- windGlobal: bool
- x: float
- y: float
Stores the current pose for a physics constraint. A physics constraint applies physics to bones. See Physics constraints in the Spine User Guide.
- PhysicsConstraint Properties
- bone: Bone
- The bone constrained by this physics constraint.
- damping: float
- data: PhysicsConstraintData readonly
- The physics constraint's setup pose data.
- gravity: float
- inertia: float
- massInverse: float
- mix: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained poses.
- strength: float
- wind: float
- PhysicsConstraint Methods
isActive ( ): bool reset ( ): void rotate ( float x, float y, float degrees): void - Rotates the physics constraint so next update forces are applied as if the bone rotated around the specified point in world space.
setToSetupPose ( ): void translate ( float x, float y): void - Translates the physics constraint so next update forces are applied as if the bone moved an additional amount in world space.
update ( Physics physics): void - Applies the constraint to the constrained bones.
Stores the setup pose for a TransformConstraint. See Transform constraints in the Spine User Guide.
- TransformConstraintData Properties
- bones: list<BoneData> readonly
- The bones that will be modified by this transform constraint.
- local: bool
- mixRotate: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained rotation.
- mixScaleX: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained scale X.
- mixScaleY: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained scale Y.
- mixShearY: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained shear Y.
- mixX: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained translation X.
- mixY: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained translation Y.
- name: string readonly, from ConstraintData
- The constraint's name, which is unique across all constraints in the skeleton of the same type.
- offsetRotation: float
- An offset added to the constrained bone rotation.
- offsetScaleX: float
- An offset added to the constrained bone scaleX.
- offsetScaleY: float
- An offset added to the constrained bone scaleY.
- offsetShearY: float
- An offset added to the constrained bone shearY.
- offsetX: float
- An offset added to the constrained bone X translation.
- offsetY: float
- An offset added to the constrained bone Y translation.
- order: int from ConstraintData
- The ordinal of this constraint for the order a skeleton's constraints will be applied by updateWorldTransform.
- relative: bool
- skinRequired: bool from ConstraintData
- When true, updateWorldTransform only updates this constraint if the skin contains this constraint. See constraints.
- target: BoneData
- The target bone whose world transform will be copied to the constrained bones.
Stores the current pose for a transform constraint. A transform constraint adjusts the world transform of the constrained bones to match that of the target bone. See Transform constraints in the Spine User Guide.
- TransformConstraint Properties
- bones: list<Bone> readonly
- The bones that will be modified by this transform constraint.
- data: TransformConstraintData readonly
- The transform constraint's setup pose data.
- mixRotate: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained rotation.
- mixScaleX: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained scale X.
- mixScaleY: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained scale X.
- mixShearY: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained shear Y.
- mixX: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained translation X.
- mixY: float
- A percentage (0-1) that controls the mix between the constrained and unconstrained translation Y.
- target: Bone
- The target bone whose world transform will be copied to the constrained bones.
- TransformConstraint Methods
isActive ( ): bool setToSetupPose ( ): void update ( Physics physics): void - Applies the constraint to the constrained bones.
- EventData Properties
- audioPath: string
- balance: float
- floatValue: float
- The event's float value.
- intValue: int
- The event's int value.
- name: string readonly
- The name of the event, which is unique across all events in the skeleton.
- stringValue: string
- The event's string value or an empty string.
- volume: float
Stores the current pose values for an Event. See Timeline apply, AnimationStateListener event, and Events in the Spine User Guide.
- Event Properties
- balance: float
- data: EventData readonly
- The events's setup pose data.
- floatValue: float
- The event's float value.
- intValue: int
- The event's int value.
- stringValue: string
- The event's string value or an empty string.
- time: float readonly
- The animation time this event was keyed.
- volume: float
- Sequence Properties
- digits: int
- id: int readonly
- Returns a unique ID for this attachment.
- regions: TextureRegion[] readonly
- setupIndex: int
- The index of the region to show for the setup pose.
- start: int
- Sequence Methods
apply ( Slot slot, HasTextureRegion attachment): void getPath ( string basePath, int index): string
Collects each visible BoundingBoxAttachment and computes the world vertices for its polygon. The polygon vertices are provided along with convenience methods for doing hit detection.
- SkeletonBounds Properties
- boundingBoxes: list<BoundingBoxAttachment> readonly
- The visible bounding boxes.
- height: float readonly
- The height of the axis aligned bounding box.
- maxX: float readonly
- The right edge of the axis aligned bounding box.
- maxY: float readonly
- The top edge of the axis aligned bounding box.
- minX: float readonly
- The left edge of the axis aligned bounding box.
- minY: float readonly
- The bottom edge of the axis aligned bounding box.
- polygons: list<float[]> readonly
- The world vertices for the bounding box polygons.
- width: float readonly
- The width of the axis aligned bounding box.
- SkeletonBounds Methods
aabbContainsPoint ( float x, float y): bool - Returns true if the axis aligned bounding box contains the point.
aabbIntersectsSegment ( float x1, float y1, float x2, float y2): bool - Returns true if the axis aligned bounding box intersects the line segment.
aabbIntersectsSkeleton ( SkeletonBounds bounds): bool - Returns true if the axis aligned bounding box intersects the axis aligned bounding box of the specified bounds.
containsPoint ( float[] polygon, float x, float y): bool - Returns true if the polygon contains the point.
containsPoint ( float x, float y): BoundingBoxAttachment - Returns the first bounding box attachment that contains the point, or null. When doing many checks, it is usually more efficient to only call this method if aabbContainsPoint returns true.
<return>
May be null.
getPolygon ( BoundingBoxAttachment boundingBox): float[] - Returns the polygon for the specified bounding box, or null.
<return>
May be null.
intersectsSegment ( float[] polygon, float x1, float y1, float x2, float y2): bool - Returns true if the polygon contains any part of the line segment.
intersectsSegment ( float x1, float y1, float x2, float y2): BoundingBoxAttachment - Returns the first bounding box attachment that contains any part of the line segment, or null. When doing many checks, it is usually more efficient to only call this method if aabbIntersectsSegment returns true.
<return>
May be null.
update ( Skeleton skeleton, bool updateAabb): void - Clears any previous polygons, finds all visible bounding box attachments, and computes the world vertices for each bounding box's polygon.
updateAabb
If true, the axis aligned bounding box containing all the polygons is computed. If false, the SkeletonBounds AABB methods will always return true.
Stores the setup pose and all of the stateless data for a skeleton. See Data objects in the Spine Runtimes Guide.
- SkeletonData Properties
- animations: list<Animation> readonly
- The skeleton's animations.
- audioPath: string
- The path to the audio directory as defined in Spine, or null if nonessential data was not exported.
- bones: list<BoneData> readonly
- The skeleton's bones, sorted parent first. The root bone is always the first bone.
- defaultSkin: Skin
- The skeleton's default skin. By default this skin contains all attachments that were not in a skin in Spine. See getAttachment.
- events: list<EventData> readonly
- The skeleton's events.
- fps: float
- The dopesheet FPS in Spine, or zero if nonessential data was not exported.
- hash: string
- The skeleton data hash. This value will change if any of the skeleton data has changed.
- height: float
- The height of the skeleton's axis aligned bounding box in the setup pose.
- ikConstraints: list<IkConstraintData> readonly
- The skeleton's IK constraints.
- imagesPath: string
- The path to the images directory as defined in Spine, or null if nonessential data was not exported.
- name: string
- The skeleton's name, which by default is the name of the skeleton data file when possible, or null when a name hasn't been set.
- pathConstraints: list<PathConstraintData> readonly
- The skeleton's path constraints.
- physicsConstraints: list<PhysicsConstraintData> readonly
- The skeleton's physics constraints.
- referenceScale: float
- Baseline scale factor for applying physics and other effects based on distance to non-scalable properties, such as angle or scale. Default is 100.
- skins: list<Skin> readonly
- All skins, including the default skin.
- slots: list<SlotData> readonly
- The skeleton's slots in the setup pose draw order.
- transformConstraints: list<TransformConstraintData> readonly
- The skeleton's transform constraints.
- version: string
- The Spine version used to export the skeleton data, or null.
- width: float
- The width of the skeleton's axis aligned bounding box in the setup pose.
- x: float
- The X coordinate of the skeleton's axis aligned bounding box in the setup pose.
- y: float
- The Y coordinate of the skeleton's axis aligned bounding box in the setup pose.
- SkeletonData Methods
findAnimation ( string animationName): Animation - Finds an animation by comparing each animation's name. It is more efficient to cache the results of this method than to call it multiple times.
<return>
May be null.
findBone ( string boneName): BoneData - Finds a bone by comparing each bone's name. It is more efficient to cache the results of this method than to call it multiple times.
<return>
May be null.
findEvent ( string eventDataName): EventData - Finds an event by comparing each events's name. It is more efficient to cache the results of this method than to call it multiple times.
<return>
May be null.
findIkConstraint ( string constraintName): IkConstraintData - Finds an IK constraint by comparing each IK constraint's name. It is more efficient to cache the results of this method than to call it multiple times.
<return>
May be null.
findPathConstraint ( string constraintName): PathConstraintData - Finds a path constraint by comparing each path constraint's name. It is more efficient to cache the results of this method than to call it multiple times.
<return>
May be null.
findPhysicsConstraint ( string constraintName): PhysicsConstraintData - Finds a physics constraint by comparing each physics constraint's name. It is more efficient to cache the results of this method than to call it multiple times.
<return>
May be null.
findSkin ( string skinName): Skin - Finds a skin by comparing each skin's name. It is more efficient to cache the results of this method than to call it multiple times.
<return>
May be null.
findSlot ( string slotName): SlotData - Finds a slot by comparing each slot's name. It is more efficient to cache the results of this method than to call it multiple times.
<return>
May be null.
findTransformConstraint ( string constraintName): TransformConstraintData - Finds a transform constraint by comparing each transform constraint's name. It is more efficient to cache the results of this method than to call it multiple times.
<return>
May be null.
Stores the current pose for a skeleton. See Instance objects in the Spine Runtimes Guide.
- Skeleton Properties
- bones: list<Bone> readonly
- The skeleton's bones, sorted parent first. The root bone is always the first bone.
- color: Color
- The color to tint all the skeleton's attachments.
- data: SkeletonData readonly
- The skeleton's setup pose data.
- drawOrder: list<Slot>
- The skeleton's slots in the order they should be drawn. The returned array may be modified to change the draw order.
- ikConstraints: list<IkConstraint> readonly
- The skeleton's IK constraints.
- pathConstraints: list<PathConstraint> readonly
- The skeleton's path constraints.
- physicsConstraints: list<PhysicsConstraint> readonly
- The skeleton's physics constraints.
- rootBone: Bone readonly
- The root bone, or null if the skeleton has no bones.
- scaleX: float
- Scales the entire skeleton on the X axis. Bones that do not inherit scale are still affected by this property.
- scaleY: float
- Scales the entire skeleton on the Y axis. Bones that do not inherit scale are still affected by this property.
- skin: Skin
- The skeleton's current skin.
- slots: list<Slot> readonly
- The skeleton's slots.
- time: float
- The skeleton's time. This is used for time-based manipulations, such as PhysicsConstraint. See update.
- transformConstraints: list<TransformConstraint> readonly
- The skeleton's transform constraints.
- x: float
- Sets the skeleton X position, which is added to the root bone worldX position. Bones that do not inherit translation are still affected by this property.
- y: float
- Sets the skeleton Y position, which is added to the root bone worldY position. Bones that do not inherit translation are still affected by this property.
- Skeleton Methods
findBone ( string boneName): Bone - Finds a bone by comparing each bone's name. It is more efficient to cache the results of this method than to call it repeatedly.
<return>
May be null.
findIkConstraint ( string constraintName): IkConstraint - Finds an IK constraint by comparing each IK constraint's name. It is more efficient to cache the results of this method than to call it repeatedly.
<return>
May be null.
findPathConstraint ( string constraintName): PathConstraint - Finds a path constraint by comparing each path constraint's name. It is more efficient to cache the results of this method than to call it repeatedly.
<return>
May be null.
findPhysicsConstraint ( string constraintName): PhysicsConstraint - Finds a physics constraint by comparing each physics constraint's name. It is more efficient to cache the results of this method than to call it repeatedly.
<return>
May be null.
findSlot ( string slotName): Slot - Finds a slot by comparing each slot's name. It is more efficient to cache the results of this method than to call it repeatedly.
<return>
May be null.
findTransformConstraint ( string constraintName): TransformConstraint - Finds a transform constraint by comparing each transform constraint's name. It is more efficient to cache the results of this method than to call it repeatedly.
<return>
May be null.
getAttachment ( int slotIndex, string attachmentName): Attachment - Finds an attachment by looking in the skin and defaultSkin using the slot index and attachment name. First the skin is checked and if the attachment was not found, the default skin is checked. See Runtime skins in the Spine Runtimes Guide.
<return>
May be null.
getAttachment ( string slotName, string attachmentName): Attachment - Finds an attachment by looking in the skin and defaultSkin using the slot name and attachment name. See getAttachment.
<return>
May be null.
getBounds ( 2-tuple offset, 2-tuple size, float[] temp, SkeletonClipping
clipper): void- Returns the axis aligned bounding box (AABB) of the region and mesh attachments for the current pose. Optionally applies clipping.
offset
An output value, the distance from the skeleton origin to the bottom left corner of the AABB.size
An output value, the width and height of the AABB.temp
Working memory to temporarily store attachments' computed world vertices.clipper
SkeletonClipping
to use. Ifnull
, no clipping is applied.
getBounds ( 2-tuple offset, 2-tuple size, float[] temp): void - Returns the axis aligned bounding box (AABB) of the region and mesh attachments for the current pose.
offset
An output value, the distance from the skeleton origin to the bottom left corner of the AABB.size
An output value, the width and height of the AABB.temp
Working memory to temporarily store attachments' computed world vertices.
physicsRotate ( float x, float y, float degrees): void - Calls rotate for each physics constraint.
physicsTranslate ( float x, float y): void - Calls translate for each physics constraint.
setAttachment ( string slotName, string attachmentName): void - A convenience method to set an attachment by finding the slot with findSlot, finding the attachment with getAttachment, then setting the slot's attachment.
attachmentName
May be null to clear the slot's attachment.
setBonesToSetupPose ( ): void - Sets the bones and constraints to their setup pose values.
setColor ( float r, float g, float b, float a): void - A convenience method for setting the skeleton color. The color can also be set by modifying color.
setScale ( float scaleX, float scaleY): void - Scales the entire skeleton on the X and Y axes. Bones that do not inherit scale are still affected by this property.
setSkin ( Skin newSkin): void - Sets the skin used to look up attachments before looking in the defaultSkin. If the skin is changed, updateCache is called. Attachments from the new skin are attached if the corresponding attachment from the old skin was attached. If there was no old skin, each slot's setup mode attachment is attached from the new skin. After changing the skin, the visible attachments can be reset to those attached in the setup pose by calling setSlotsToSetupPose. Also, often apply is called before the next time the skeleton is rendered to allow any attachment keys in the current animation(s) to hide or show attachments from the new skin.
newSkin
May be null.
setSkin ( string skinName): void - Sets a skin by name. See setSkin.
setSlotsToSetupPose ( ): void - Sets the slots and draw order to their setup pose values.
setToSetupPose ( ): void - Sets the bones, constraints, slots, and draw order to their setup pose values.
update ( float delta): void - Increments the skeleton's time.
updateCache ( ): void - Caches information about bones and constraints. Must be called if the skin is modified or if bones, constraints, or weighted path attachments are added or removed.
updateWorldTransform ( Physics physics, Bone parent): void - Temporarily sets the root bone as a child of the specified bone, then updates the world transform for each bone and applies all constraints. See World transforms in the Spine Runtimes Guide.
updateWorldTransform ( Physics physics): void - Updates the world transform for each bone and applies all constraints. See World transforms in the Spine Runtimes Guide.
Determines how physics and other non-deterministic updates are applied.
- Physics Values
- none
- Physics are not updated or applied.
- reset
- Physics are reset to the current pose.
- update
- Physics are updated and the pose from physics is applied.
- pose
- Physics are not updated but the pose from physics is applied.
Base class for loading skeleton data from a file. See JSON and binary data in the Spine Runtimes Guide.
- SkeletonLoader Properties
- scale: float
- Scales bone positions, image sizes, and translations as they are loaded. This allows different size images to be used at runtime than were used in Spine. See Scaling in the Spine Runtimes Guide.
- SkeletonLoader Methods
readSkeletonData ( object data): SkeletonData - Deserializes the Spine skeleton data into a SkeletonData object.
SkeletonBinary
extends SkeletonLoaderLoads skeleton data in the Spine binary format. See Spine binary format and JSON and binary data in the Spine Runtimes Guide.
- SkeletonBinary Properties
- BONE_ROTATE = 0: int static, readonly
- BONE_TRANSLATE = 1: int static, readonly
- BONE_TRANSLATEX = 2: int static, readonly
- BONE_TRANSLATEY = 3: int static, readonly
- BONE_SCALE = 4: int static, readonly
- BONE_SCALEX = 5: int static, readonly
- BONE_SCALEY = 6: int static, readonly
- BONE_SHEAR = 7: int static, readonly
- BONE_SHEARX = 8: int static, readonly
- BONE_SHEARY = 9: int static, readonly
- BONE_INHERIT = 10: int static, readonly
- SLOT_ATTACHMENT = 0: int static, readonly
- SLOT_RGBA = 1: int static, readonly
- SLOT_RGB = 2: int static, readonly
- SLOT_RGBA2 = 3: int static, readonly
- SLOT_RGB2 = 4: int static, readonly
- SLOT_ALPHA = 5: int static, readonly
- ATTACHMENT_DEFORM = 0: int static, readonly
- ATTACHMENT_SEQUENCE = 1: int static, readonly
- PATH_POSITION = 0: int static, readonly
- PATH_SPACING = 1: int static, readonly
- PATH_MIX = 2: int static, readonly
- PHYSICS_INERTIA = 0: int static, readonly
- PHYSICS_STRENGTH = 1: int static, readonly
- PHYSICS_DAMPING = 2: int static, readonly
- PHYSICS_MASS = 4: int static, readonly
- PHYSICS_WIND = 5: int static, readonly
- PHYSICS_GRAVITY = 6: int static, readonly
- PHYSICS_MIX = 7: int static, readonly
- PHYSICS_RESET = 8: int static, readonly
- CURVE_LINEAR = 0: int static, readonly
- CURVE_STEPPED = 1: int static, readonly
- CURVE_BEZIER = 2: int static, readonly
- scale: float from SkeletonLoader
- Scales bone positions, image sizes, and translations as they are loaded. This allows different size images to be used at runtime than were used in Spine. See Scaling in the Spine Runtimes Guide.
- SkeletonBinary Methods
readSkeletonData ( object data): SkeletonData from SkeletonLoader - Deserializes the Spine skeleton data into a SkeletonData object.
SkeletonJson
extends SkeletonLoaderLoads skeleton data in the Spine JSON format. JSON is human readable but the binary format is much smaller on disk and faster to load. See SkeletonBinary. See Spine JSON format and JSON and binary data in the Spine Runtimes Guide.
- SkeletonJson Properties
- scale: float from SkeletonLoader
- Scales bone positions, image sizes, and translations as they are loaded. This allows different size images to be used at runtime than were used in Spine. See Scaling in the Spine Runtimes Guide.
- SkeletonJson Methods
readSkeletonData ( object data): SkeletonData from SkeletonLoader - Deserializes the Spine skeleton data into a SkeletonData object.
Stores attachments by slot index and attachment name. See SkeletonData defaultSkin, Skeleton skin, and Runtime skins in the Spine Runtimes Guide.
- Skin Properties
- attachments: list<SkinEntry> readonly
- Returns all attachments in this skin.
- bones: list<BoneData> readonly
- color: Color
- The color of the skin as it was in Spine, or a default color if nonessential data was not exported.
- constraints: list<ConstraintData> readonly
- name: string readonly
- The skin's name, which is unique across all skins in the skeleton.
- Skin Methods
addSkin ( Skin skin): void - Adds all attachments, bones, and constraints from the specified skin to this skin.
clear ( ): void - Clears all attachments, bones, and constraints.
copySkin ( Skin skin): void - Adds all bones and constraints and copies of all attachments from the specified skin to this skin. Mesh attachments are not copied, instead a new linked mesh is created. The attachment copies can be modified without affecting the originals.
getAttachment ( int slotIndex, string name): Attachment - Returns the attachment for the specified slot index and name, or null.
<return>
May be null.
getAttachments ( int slotIndex, list<SkinEntry> attachments): void - Returns all attachments in this skin for the specified slot index.
removeAttachment ( int slotIndex, string name): void - Removes the attachment in the skin for the specified slot index and name, if any.
setAttachment ( int slotIndex, string name, Attachment attachment): void - Adds an attachment to the skin for the specified slot index and name.
Stores an entry in the skin consisting of the slot index and the attachment name.
- SkinEntry Properties
- name: string readonly
- The name the attachment is associated with, equivalent to the skin placeholder name in the Spine editor.
- slotIndex: int readonly
Stores the setup pose for a Slot.
- SlotData Properties
- attachmentName: string
- The name of the attachment that is visible for this slot in the setup pose, or null if no attachment is visible.
- blendMode: BlendMode
- The blend mode for drawing the slot's attachment.
- boneData: BoneData readonly
- The bone this slot belongs to.
- color: Color
- The color used to tint the slot's attachment. If darkColor is set, this is used as the light color for two color tinting.
- darkColor: Color
- The dark color used to tint the slot's attachment for two color tinting, or null if two color tinting is not used. The dark color's alpha is not used.
- index: int readonly
- The index of the slot in slots.
- name: string readonly
- The name of the slot, which is unique across all slots in the skeleton.
- visible: bool
- False if the slot was hidden in Spine and nonessential data was exported. Does not affect runtime rendering.
Determines how images are blended with existing pixels when drawn.
Stores a slot's current pose. Slots organize attachments for drawOrder purposes and provide a place to store state for an attachment. State cannot be stored in an attachment itself because attachments are stateless and may be shared across multiple skeletons.
- Slot Properties
- attachment: Attachment
- The current attachment for the slot, or null if the slot has no attachment.
- bone: Bone readonly
- The bone this slot belongs to.
- color: Color
- The color used to tint the slot's attachment. If darkColor is set, this is used as the light color for two color tinting.
- darkColor: Color
- The dark color used to tint the slot's attachment for two color tinting, or null if two color tinting is not used. The dark color's alpha is not used.
- data: SlotData readonly
- The slot's setup pose data.
- deform: float[]
- Values to deform the slot's attachment. For an unweighted mesh, the entries are local positions for each vertex. For a weighted mesh, the entries are an offset for each vertex which will be added to the mesh's local vertex positions. See computeWorldVertices and DeformTimeline.
- sequenceIndex: int
- The index of the texture region to display when the slot's attachment has a Sequence. -1 represents the setupIndex.
- skeleton: Skeleton readonly
- The skeleton this slot belongs to.
- Slot Methods
setToSetupPose ( ): void - Sets this slot to the setup pose.